My form first just had the HTML in the form label as so:
from django import forms class AccountForm(forms.Form): name = forms.CharField(widget=forms.TextInput(), max_length=15, label='Your Name (why?')
However, when I displayed it, the form was autoescaped.
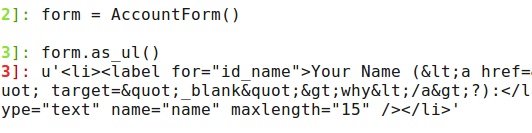
This is generally a good thing, except my form obviously didn't display correctly. I tried autoescaping it in the template, but that didn't work. To resolve this you'll need to mark that individual label as safe. Thus:
from django.utils.safestring import mark_safe from django import forms class AccountForm(forms.Form): name = forms.CharField(widget=forms.TextInput(), max_length=15, label=mark_safe('Your Name (why?)'))
It will now display correctly:
In [1]: from myproject.forms import * In [2]: form = AccountForm() In [3]: form.as_ul() Out[3]: u'
There's maybe another easier way to do this, but this worked for me.